Remote Controller test
A few years back we bought a Pi Hut wireless controller at the fantastic Raspberry Fields, which I’m sad to say that despite the varied amount of buttons and joysticks it contains we haven’t used in a single project since.
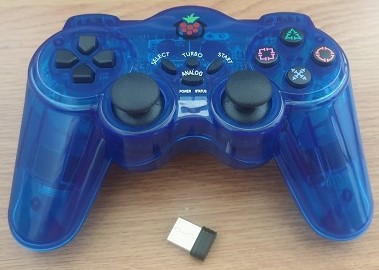
The test setup looked like this. The Pi is connected to a USB hub containing a wireless mouse, keyboard and finally the Controller.
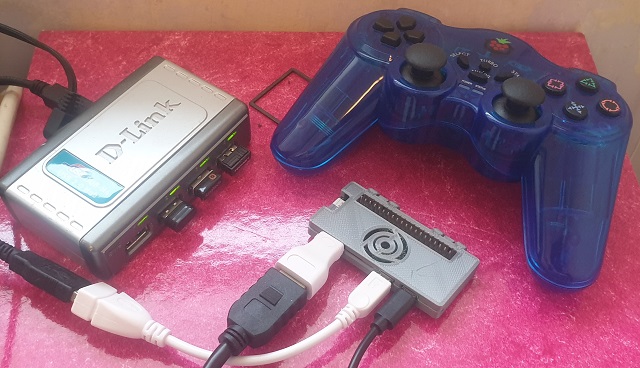
Following instructions from the Pi Hut page, it points the the Approximate Engineering’s Python Game Controller library built for a PiWars robot. Installation was simple so I headed over to the Simple Usage section to try things out and built some python to connect to the controller.
Using some Python similar to the right built from the examples looked to be working, but didn’t seem to register any of the controller button presses.
It also had the problem of not being able to escape and sleep obviously not being imported!
from approxeng.input.selectbinder import ControllerResource
while True:
try:
with ControllerResource() as joystick:
print('Found a joystick and connected')
while joystick.connected:
# Do stuff with your joystick here!
# ....
# ....
# If we had any presses, print the list of pressed buttons by standard name
if joystick.has_presses:
print(joystick.presses)
# Joystick disconnected...
print('Connection to joystick lost')
except IOError:
# No joystick found, wait for a bit before trying again
print('Unable to find any joysticks')
sleep(1.0)
from approxeng.input.controllers import find_matching_controllers
from approxeng.input.controllers import print_controllers
from approxeng.input.controllers import print_devices
discoveries = find_matching_controllers()
print_controllers()
print_devices()
In trying to figure out why it was connecting but not registering any key presses I looked to see what else it could be picking up.
This seems to pick up a number of controllers / devices, so I can only assume the code picked the first one it found and this wasn’t the correct one.
On the hunt for further examples to test led to the “Examples” section. One that jumped out and looked fairly simple was “Streaming Axis and Button Hold values”. I tried this and it worked!
The only difference was the line:
ControllerRequirement(require_snames=['lx', 'ly'])
which in fairness an early example in the simple usage section did list:
with ControllerResource(ControllerRequirement(require_class=DualShock3),
ControllerRequirement(require_snames=['lx','ly'])) as ds3, joystick:
This allows it to pick the first device that has a left joystick. so adding this and rerunning now worked!
pi@carpizero:~/code/TheLemmingFarm/Code/controller $ python3 checkbuttons.py
Found a joystick and connected
Left stick: x=0, y=0 Right stick: x=0, y=0
Left stick: x=0, y=0 Right stick: x=0, y=0
Left stick: x=1.0, y=0.3850157346889374 Right stick: x=0, y=0
Left stick: x=0, y=1.0 Right stick: x=0, y=0
Left stick: x=-1.0, y=0 Right stick: x=0, y=0
['r2']
Left stick: x=0, y=0 Right stick: x=0, y=0
Left stick: x=0, y=0 Right stick: x=0, y=0
['l2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l1']
Left stick: x=0, y=0 Right stick: x=0, y=0
['r1']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l1']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l2', 'r1', 'r2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l1', 'r1', 'r2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l1', 'r1', 'r2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['l1', 'r1']
Left stick: x=0, y=0 Right stick: x=0, y=0
['r2']
Left stick: x=0, y=0 Right stick: x=0, y=0
['select']
Left stick: x=0, y=0 Right stick: x=0, y=0
^CStopping Joystick test
Now the comms and ability to read inputs has been proven the more advanced examples can be checked. To fit in with existing code Id like to use button call-backs and the failover example to reconnect if it drops out of range.